반응형
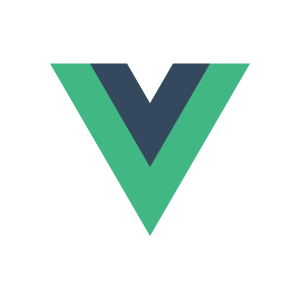
Vue에서 Intl API를 사용하여 다국어 기능을 구현하는 방법은 다음과 같습니다.
1. 필요한 패키지 설치:
- vue-i18n 패키지를 설치합니다. 이 패키지는 Vue 컴포넌트에서 Intl API를 쉽게 사용할 수 있도록 도와줍니다.
npm install vue-i18n
2. 번역 파일 준비:
- src/locales 디렉토리를 생성합니다.
- 각 언어 코드에 해당하는 번역 파일을 [언어 코드].json 형식으로 생성합니다.
- 예를 들어, 한국어 번역 파일은 ko.json 이름으로 생성하고 다음과 같은 내용을 포함합니다.
{ "message": "안녕하세요, Vue Intl!", "welcome": "환영합니다!" }
3. Vue I18n 인스턴스 설정:
- main.js 또는 App.vue 파일에서 Vue I18n 인스턴스를 설정합니다.
import Vue from 'vue'; import VueI18n from 'vue-i18n'; import ko from './locales/ko.json'; import en from './locales/en.json'; Vue.use(VueI18n); const i18n = new VueI18n({ locale: 'ko', // 기본 언어 설정 messages: { ko: ko, en: en } }); new Vue({ i18n, // ... 컴포넌트 코드 }).$mount('#app');
4. Intl 사용하기
common/global.js
const i18n_intl = {
/**
*
* @param {string} style 'time' or 'date'
* @param {string} format 'full', 'long', 'medium', 'short'
* @returns {Intl.DateTimeFormat}
*/
getFormatter(style, format) {
if (typeof format !== 'string' || !['full', 'long', 'medium', 'short'].includes(format)) {
throw new Error('format Error')
}
return new Intl.DateTimeFormat('ko', { [style]: format })
},
getRelativeTimeFormat: new Intl.RelativeTimeFormat('ko', { numeric: 'auto' }),
/**
*
* @param {Number} number
* @returns String
*/
getCommaFormat(number) {
return new Intl.NumberFormat('ko', { style: 'decimal', maximumFractionDigits: 0 }).format(number)
},
/**
*
* @param {Number} number
* @returns String
*/
getPercentFormat(number) {
return new Intl.NumberFormat('ko', { style: 'percent' }).format(number)
},
}
const now = new Date()
export const intl = {
fullTimeFormat: i18n_intl.getFormatter('time', 'full').format(now),
longTimeFormat: i18n_intl.getFormatter('time', 'long').format(now),
mediumTimeFormat: i18n_intl.getFormatter('time', 'medium').format(now),
shortTimeFormat: i18n_intl.getFormatter('time', 'short').format(now),
fullDateTimeFormat: i18n_intl.getFormatter('datetime', 'full').format(now),
longDateTimeFormat: i18n_intl.getFormatter('datetime', 'long').format(now),
mediumDateTimeFormat: i18n_intl.getFormatter('datetime', 'medium').format(now).slice(0, -1),
shortDateTimeFormat: i18n_intl.getFormatter('datetime', 'short').format(now).slice(0, -1),
dayBeforeDateFormat: i18n_intl.getRelativeTimeFormat.format(-2, 'day'),
yesterdayDateFormat: i18n_intl.getRelativeTimeFormat.format(-1, 'day'),
todayDateFormat: i18n_intl.getRelativeTimeFormat.format(0, 'day'),
tomorrowDateFormat: i18n_intl.getRelativeTimeFormat.format(1, 'day'),
dayAfterTomorrowDateFormat: i18n_intl.getRelativeTimeFormat.format(2, 'day'),
lastWeekDateFormat: i18n_intl.getRelativeTimeFormat.format(-1, 'week'),
weekDateFormat: i18n_intl.getRelativeTimeFormat.format(0, 'week'),
nextWeekDateFormat: i18n_intl.getRelativeTimeFormat.format(1, 'week'),
lastMonthDateFormat: i18n_intl.getRelativeTimeFormat.format(-1, 'month'),
monthDateFormat: i18n_intl.getRelativeTimeFormat.format(0, 'month'),
nextMonthDateFormat: i18n_intl.getRelativeTimeFormat.format(1, 'month'),
commaFormat: i18n_intl.getCommaFormat,
percentFormat: i18n_intl.getPercentFormat,
}
import { intl } from '@/common/global'
intl.commaFormat(1234567.89) // 1,234,568
intl.commaFormat(100000) // 100,000
intl.commaFormat(823749827) // 823,749,827
intl.percentFormat(1) // 100%
intl.percentFormat(0.7) // 70%
intl.percentFormat(0.71) // 71%
intl.percentFormat(1 / 4) // 25%
intl.dayBeforeDateFormat // 그저께
intl.yesterdayDateFormat // 어제
intl.todayDateFormat // 오늘
intl.tomorrowDateFormat // 내일
intl.dayAfterTomorrowDateFormat // 모레
intl.lastWeekDateFormat // 지난주
intl.weekDateFormat // 이번 주
intl.nextWeekDateFormat // 다음 주
intl.lastMonthDateFormat // 지난달
intl.monthDateFormat // 이번 달
intl.nextMonthDateFormat // 다음 달
intl.fullDateTimeFormat // 2024년 7월 18일 목요일
intl.longDateTimeFormat // 2024년 7월 18일
intl.mediumDateTimeFormat // 2024. 7. 18
intl.shortDateTimeFormat // 24. 7. 18
intl.fullTimeFormat // 오후 5시 58분 45초 한국 표준시
intl.longTimeFormat // 오후 5시 58분 45초 GMT+9
intl.mediumTimeForma // 오후 5:58:45
intl.shortTimeFormat // 오후 5:58
Vue I18n
Vue I18n Internationalization plugin for Vue.js Easy, powerful, and component-oriented for Vue.js
vue-i18n.intlify.dev
https://velog.io/@seo__namu/JavaScript-Intl-API-%EC%82%AC%EC%9A%A9%ED%95%98%EA%B8%B0
JavaScript Intl API 사용하기
Intl API를 사용해서 시간 똑똑하게 나타내기
velog.io
728x90
반응형
'웹개발 > vuejs' 카테고리의 다른 글
Vue 3 및 Router 4 기초부터 상세 가이드 (4) | 2024.07.20 |
---|---|
[Vue] Vue3에서 새창 띄우기 (0) | 2024.07.19 |
[Vue] Vue3에서 setup() 함수와 함께 Axios와 Async/Await 사용하기 (1) | 2024.07.16 |
[Vue] Vue2에서 Axios와 함께 Async/Await 사용하기 (0) | 2024.07.16 |
[Vue] Vite에서 환경변수 env 사용하기 (0) | 2024.07.16 |